In this tutorial, you will learn how to use JCheckBox class to create checkboxes.
To create checkboxes, you use the JCheckBox class. The JCheckBox class inherits all functionality of JToogleButton class. A checkbox allows the user to turn something on and off. You can create a checkbox with text, icon or both. Here are the most typical constructors of JCheckBox class:
JCheckBox Constructors | Meanings |
---|---|
public JCheckBox( ) | Creates a checkbox without text and icon. The state of the checkbox is not selected. |
public JCheckBox(Icon icon) | Creates a checkbox with icon |
public JCheckBox(Icon icon, boolean selected) | Creates a checkbox with icon and initialize the state of checkbox by the boolean parameter selected |
public JCheckBox(String text) | Creates a checkbox with text |
public JCheckBox(String text, boolean selected) | Creates a checkbox with text and initialize the state of the checkbox |
public JCheckBox(String text, Icon icon) | Creates a checkbox which displays both text and icon. |
public JCheckBox(String text, Icon icon, boolean selected) | Creates a checkbox which displays both text and icon. The state of the checkbox can be initialized. |
There are two ways to work with checkbox’s state:
- You use addActionListener() or AddItemListener() method so that whenever the state of checkbox change you can have corresponding logic to handle this.
- Use isSelected() to check whether the checkbox is selected.
JCheckbox Demo Application
In this example, we create a checkbox. When the user clicks on it, a message will display to notify the checkbox’s state. Here is the output of the demo application:
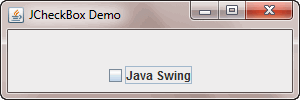
When user checks the checkbox, a message popup will display like the following screenshot:
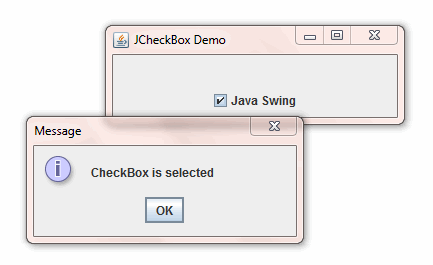
Here is the code sample of JCheckBox demo:
package jcheckboxdemo;
import java.awt.BorderLayout;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import javax.swing.JCheckBox;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
public class Main {
public static void main(String[] args) {
final JFrame frame = new JFrame("JCheckBox Demo");
JCheckBox chkSwing = new JCheckBox("Java Swing");
chkSwing.addItemListener(
new ItemListener() {
public void itemStateChanged(ItemEvent e) {
if (e.getStateChange() == ItemEvent.SELECTED) {
JOptionPane.showMessageDialog(frame,
"CheckBox is selected");
} else {
JOptionPane.showMessageDialog(frame,
"CheckBox is unselected");
}
}
});
// Add checkbox to a panel
JPanel buttonPanel = new JPanel();
buttonPanel.add(chkSwing);
// Frame setting
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 100);
frame.getContentPane().add(buttonPanel, BorderLayout.SOUTH);
frame.setVisible(true);
}
}
Code language: JavaScript (javascript)