In this tutorial, you will learn how to use JToggleButton class to create toggle buttons in Swing.
A toggle button is a two-state button that allows the user to switch on and off. To create a toggle button in Swing you use JToggleButton class.
Here are the most common used constructors of the JToggleButton class:
JToggleButton Constructors | Meanings |
public JToggleButton( ) | Creates a toggle button without text and icon. The state of the toggle button is not selected. |
public JToggleButton(Icon icon) | Creates a toggle button with icon |
public JToggleButton(Icon icon, boolean selected) | Creates a toggle button with icon and initialize the state of toggle button by the boolean parameter selected |
public JToggleButton(String text) | Creates a toggle button with text |
public JToggleButton(String text, boolean selected) | Creates a toggle button with text and initialize the state of the toggle button |
public JToggleButton(String text, Icon icon) | Creates a toggle button which displays both text and icon. |
public JToggleButton(String text, Icon icon, boolean selected) | Creates a toggle button which displays both text and icon. The state of toggle button can be initialized. |
Example of using JToggleButton
In this example, we will create a simple toggle button by using the JToggleButton class. We will also add different event handlers to handle click event. A popup message will display whenever the user clicks the button.
Here is the screenshot of the demo application:
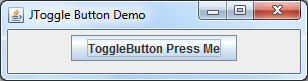
package jtogglebuttondemo; package jtogglebuttondemo; import java.awt.FlowLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.ItemEvent; import java.awt.event.ItemListener; import javax.swing.JFrame; import javax.swing.JOptionPane; import javax.swing.JToggleButton; import javax.swing.event.ChangeEvent; import javax.swing.event.ChangeListener; public class Main { public static void main(String[] args) { final JFrame frame = new JFrame("JToggle Button Demo"); frame.setSize(200, 100); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.getContentPane( ).setLayout(new FlowLayout()); JToggleButton jtbButton = new JToggleButton("ToggleButton Press Me"); jtbButton.addActionListener(new ActionListener( ) { public void actionPerformed(ActionEvent ev) { JOptionPane.showMessageDialog(frame, "Action Event" ); } }); jtbButton.addItemListener(new ItemListener( ) { public void itemStateChanged(ItemEvent ev) { JOptionPane.showMessageDialog(frame, "Item Event" ); } }); jtbButton.addChangeListener(new ChangeListener( ) { public void stateChanged(ChangeEvent ev) { JOptionPane.showMessageDialog(frame, "Change event" ); } }); frame.add(jtbButton); frame.setVisible(true); } }